I see there is a method to check if a variable is an array, but I don't see something similar to check if a variable is a string.
Is there a method for that?
If not, is there a method to check if a variable is an integer?
I would like to make an inline function that can accept either an integer or a string as one of the arguments, but they need separate handling inside the inline function.
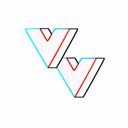
Posts
-
Quick coding question: Is there a way to check if a variable is a string?
-
RE: Is there a method to rotate a path?
@d-healey said in Is there a method to rotate a path?:
@VirtualVirgin Instead of making a function to rotate a path, why not make a function to create a rotated path. You can pass in the data you would have used to create the initial path.
So based on your suggestion I came up with this and it seems to work:
inline function createRotatedPath(pathArray, angle, area) { local rad = Math.toRadians(angle); local cosA = Math.cos(rad); local sinA = Math.sin(rad); local path = Content.createPath(); local rotationCenterX = area[0] + (area[2] * 0.5); local rotationCenterY = area[1] + (area[3] * 0.5); for (i = 0; i < pathArray.length; i++) { local x = pathArray[i][0]; local y = pathArray[i][1]; local newX = (x - rotationCenterX) * cosA - (y - rotationCenterY) * sinA + rotationCenterX; local newY = (x - rotationCenterX) * sinA + (y - rotationCenterY) * cosA + rotationCenterY; if (i == 0) path.startNewSubPath(newX, newY); else path.lineTo(newX, newY); } path.closeSubPath(); return path; }
The pathArray is a 2D array of x,y points
i.e.
[[x,y],[x,y] etc..] -
RE: Is there a method to rotate a path?
Now I'm trying to make an inline function to rotate a path:
inline function rotatePath(path, angle)
But the problem I am running into is that I cannot find any way to "unpack" the path coordinates to apply transformations to them.
Javascript has path.getPoints(), but I see no analog in HISE. -
RE: Is there a method to rotate a path?
@orange Thank you, but that rotates the canvas, not the path itself, and as I mentioned above I need to rotate the path itself in order to get the "hit zone" for mouse events in the mouse callbacks (using path.contains()).
-
Is there a method to rotate a path?
It would make a few things simpler over here if there is a method for path rotation, but I don't see anything in the docs which matches that description (such as "transform").
Is the only way to rotate by doing the trig on my original path? Just wondering if there is something more convenient.
I do know that I can rotate the canvas, but I need the actual path to rotate so I can use path.contains() for mouse callbacks. -
RE: Does path.contains() work properly? I am having issues with it.
@ustk Oh that makes sense! And that method is a lot easier than what I was doing.
-
Does path.contains() work properly? I am having issues with it.
I have a path here and I am trying to detect the mouse events inside the bounds of the path with path.contains():
UpArrowPanel.setMouseCallback(function(event) { var inBounds = arrowPath.contains([event.x, event.y]); // Handle hover state if (event.hover) inBounds ? isHovered = true : isHovered = false; etc..
But the detection I am getting is clearly off from the actual path:
Here is a snippet of the arrow path with paint routine and mouse callback:
HiseSnippet 1459.3ocsW0uaaaCDmJMpa1acaEXO.D4ujaxbscSZRVvPa9bMXMoF0oECnnnflhxhnxjBjzw0qnOY6kZuAaGojrk+HNoFXFvIl2we2c7ti2crsRRYZsTg7pd0nTFx6686LRXhONlvEnyOA48C9WPzFlBmQ5nQoDslEh77t2uaI3UYcj6y+7riHIDAkMgDB8VImxdIuO2LgZ6m+G7jjyHgrq38Ks6se94To3XYhb.XO2yuAJkP+HoG6Rhcaq4idAQGi7djOkt89r8hZsaiV6y1Ib6tsZ1fzpw96uKirSCZ28YjVQcoMPd2+zPtQp5XHFlFD5QxvQchkCEYJ3sbMuaBytnIpCn4Lxnii4IgsKbNZDxa81SbU2KyU8y9WvC4ioOwk8SNF3IHJ6z7VaYlTyuBSxqjIsdlI8P+NTEO0Lgi0d9N+yEPDLh.wlxlR1dQq82U7OVB6PXp2m7Q1YJXwXDAMa0XKL7mZGTsJDezF7aROTojCaSDrD7ugKvRBCcjB1n7F1XK71MreA7koWWyLAaLjGZh23FYGy38hM2LeRRhb3wve6BYJZXeabXRBdBgI1LwhrCkjv.Kt4A3G+Xb1J3LBQCbD7Uwz7+hK5gMwrL.kA2lXhKcZoJFD.sDCrZ4ZhBy0uPdMSwBgsEQRzrCxI2FjrtL4pbQBW.JeffZ3RANSZGVnmfZU+bUL7YrlqSSXDkSUV5IRv3wZ6YoE9QkNctS1UJNQzCNbN2aI.FKfcWBfLGdIDCADOcd.ulQMKVEw2fMMAQtNbX.FuJJBhkNednhLz5+6NBGzDR5p4BKTlMWzRmHBwFINUwtFngoI7zTfdIsS+jU86f2DhwkIOpD4BEC2.UPrETcwo2vSmwqqs64R1vNC55hKzOskUX+B3IeLtUsCJj0KYQkjCKrGaFAYC2WIA7.VsEqSNatP4n4gLrLBxGycYKQVCuYYcjzXj8wIVQtpRC9e7bRTYie2MQt4sIxW6j0c+Du4xNwYB6tEF1bYggifpG1Ls4SHnIRMqHa..7En.yr2cgTrYKU0F5MXdsbfAzePws9fdEWy6UOBZIB0tBxZ+oqOLlaX4Fz3DV3ZBE3qvcI1xIPcCssKhiOOBGLtRSMGoLICZOSnikcOEiIxkMCpGkiMu30shcTInKcu4mgrBLDgNknrWasG0oNWmADlTyEmRxKnj4Ub9zwAfsvuyVYXK7S1apZLyQ380lbS+DnthSAP.vlAT8lr3tIPfO+30qtsbzppcXW0s8r9xhxFtPNPyJ5RMIcvUUqHkv04PbjbfHzVluTFHz.BxlzAuys+5PEoreL58yju7BndosdqMtNSlRFBGmZUqTYrhd1TMwLpAL7utn9ZynBnRLbgYQpvwoHo5yiSWbYb4JcRBmiSotkV8mcj9xzIqYxtu0M9lzEJ64uILqvyOIikdkohRJVp8JaPdHbbiZo3Rog8JgqCcE35OdVVQQKjmctAkLIgoVHa6nvpkALPLneWlZKHwHY.a7FgQ+lddx6e2lmjlMFSoMJEmK3lWkxxWelLIzNmn82yO8IJeNH3Wu47SHFhcfzbZv9RYJC2ZNdmvtFdBP13oU7Ogo+nQl51a+TovJAuuw339fhgWsA.DOz91ixwDzmPd9dAfFGU7C2vGfbueP96MxlsnLkoGQD48i9SMhH7bj4lhFleWFNHgXldnd6ycxY.Qlolj1NsrPyMiJ+bnuhI8arzI8uql3C8ayMz3Eaiqs.aDhY+eXi4uO5A9mFEAMzmXfq6e1etpOF5VTeVu0dWPft+17jKGzuCTRmx.sKfjGsMkYMada15F10VOPGlHzs3egO4LaZW6kyrYASTeBUI+.M69n8EXeqiBXSB2KTq.OUFViahb2QK6m6COH7CT5zhZNfsVUfOYUAt8pBbmUE3SWUf6tp.261AZeu9gCfYayt1fPWz9TWQMOuSEDHCzksh9O.EyG8cB
-
RE: A bizarre incident:
@d-healey How do you prefer to save your projects?
-
RE: A bizarre incident:
@d-healey said in A bizarre incident::
@VirtualVirgin said in A bizarre incident::
The project opened
.hip or .xml?
.hip
-
A bizarre incident:
A bizarre incident:
I was working on a project which was compiling fine with no errors.
I had to close the project to work on some others, and later I reopened it.
It would crash trying to load every time.
The other projects do not contain any shared scripts with the one in question here (so there is no editing that would be done there which would effect the project that is crashing).
After trying to swap out a few of the .js external scripts and failing to get the project to open (just in case I left something in one of them which could cause an issue),
I then renamed the "Scripts" folder to "**Scripts" in order to try opening the project without any of the .js scripts attached (thinking HISE would not "see" any scripts). Well guess what? The project opened without any errors and was fully functional!
I checked the the project folder and the "**Scripts" folder was now gone and replaced with a "Scripts" folder.
So I am scratching my head as to what happened. HISE found my "**Scripts" folder and renamed it? And somehow that made it able to load without crashing? Very confused.
If anyone has a hypothesis, I'd love to hear it! -
RE: Viewport scrollbars are hidden if child is larger in 2 dimensions
@rglides said in Viewport scrollbars are hidden if child is larger in 2 dimensions:
A little extra code but a lot more customisability
HiseSnippet 1592.3ocuXk0aabCDlqs2fnswAM.8oBT.VihfUMN9LIs.NGp9p1nwIBQNtxHH0gdWJIBuZo5tT1Vwwn4mRAJP+Ez2i+WzWa+GzmJ5atyrGZ4pi3CzT8fs3v43aFNC4LpbfzgGFJCHFE1rSKNw3ZlU53qZrTClvmr9xDiOybSdnR3WmtkfePKYfhF5DH871kEDRVrSKVXH2kXXL52hhXTXLRzm+5QKx7X9N7LRDxVRgC+whlBUF0xk9Ngm2pLW9lhlZbemRq6H8WR5IaCvaTyYHsXN6wpyeBCYaDShwUVwUnjAUTLEOjXL1hR2NUZHOvOl+sDghc833hYIU.EESdUomKhXjJYoFBO2xoggPBnkxYAkQiCJeh4FBWQW5YAmONZCZlD5wCiQxCuQyAuY0g2LZva.PxPCRiECoaXVwIPzRksChmOxbceEOnFCB65PIlWxH+5UMWRBb3qlpIaO9pAvhtRXeuYlYRJ7mhKXYAg9PEsLym6MK8AzTopyUKIa1R5CKrmHd6I.9i+1TgbUYH1ndlrMjxvsq012QIj910KZcjUg8YATFnMUCQHppGKcXdKJa66FZiVMhg19BUEwq4.eycWflUg5ndiSDri+W3Tdh5MTNx.lGJW8opA4POi6nrYH5KL8ztArC.CwoAxCnd.XBGrh10CxpPcTSFPsEfUmYApfdeJ6Ey+xoSACP5V2pnUAvI.sf59wn+AwK1Kl6kSBB7kY.+Vc+56Yi4JtPAv2NdPFdtyzvWb6EA04eYjgQydLFmld5aet9TwS3xCnU5V3mlffGXs781IamglrjmMLoISEcouy1CUdMdFlvUOGBWEE1BkyiEp1P1Njugb+n6d.oWwuNDdQgedKEPxFXF4UDFw4S2GhBOfVi4ExuHguTqSqRY9tzssrD9XNIMs.gJ807OzGfE1NoNvjfi50liEQT3SV0l8DclXRpcBA.1qwwBC6hzogCZHWHVtErNdAKMCDWGDYjkXHMm8rGDBvP06EpUuHP8v7P86EtpFmARqdVHspNRu3mGqA40ff4yMQaFcb20hcuGiuO3hEG25nwi7NQMZLooZHwTi27lz0GReHTPSu4Mooqu+C5oRQOF.7EqwA8IVCc5QicFrFSS.JVLVgGko2yYBel.n684Zo9Ey15n7vMe8gJnMeg7LjK4CdCLMuAYs3P4s56k2ii+Zx+3PMYet7fpa6J0wnxFe.G9XrIn+C+7m65AF7To+HI81CHj+PHauOXNrnSDf0c4gEZxwXpq0qioXAwtl8cmA2Wqh9IRE+ofGhuRacrEs2spUaf6kXdOPkCZ6Xi8dDz1ucyc4AZ2YDwHzkS9VmLGdqS5c14De2uFiR+0gW.eZKt+v52ij7fAzl0HInBXUE0j00SZxJ94Oh.5kZbSsi.RDrSaY82+kSdG4BphsyohR+4q+s2Qd95KyTLrsuDnAvsEOPIvHgwx78gdniaBrf4x7v8TxVQXO4BXnKvXSOdZKh3MtQV9iMymRPNLqc6enTmtKd6KJc.dwTWB+rpTin6Ux3WUBpOfDvjpjPT6K4IfuQuIcM71Pxt0S6duvUN8zSiDSn3MynlNWPF04zIq3Gp5WE470qcNOoz7zGp4omDTJT0wKZvm0jAhWCQblGIu2+Gs5y6+hRMyMry6ZxNTePGr1bS.8KJOD6+tEK.PqFr66jX+zLZiQIWX2aaM26UgZtGLDUh6YYtElB4zmyAtRONG3t++3bWYX4oWORAoyap4bmrqlyU5UkNqyIfBVE00hEFlEupYbeIcs0ee5iJcRhsd6+b5oyehrE6GwJUiQxa0R+TuVEnzWLoGWp2ZmOMo1Yx3RGnDZ4.Vc3Ak5v.x8M7GL1ozssGSkeVTb.7jMfaYyM.HNjmenP0Qe.8+yFP87BwaXVVnbZLXLNx.vHVr+A.iIi0Ot4J0pAyLlAvwLWs5GlY3IwiDWeClJP.IYlOocyJvEaNbv59PpG9JmwH36QwqmAWiQfJbe2nEv8emlr4r3ZijMmMcSRSFTysiS7SP3ObvUin.XxO52Lof4F3Z5r4e3B+zT3J1wwIup5Sv4trBN+kUv6bYE7tWVAu2kUvu5xJ3We1Bh+LSeSakrYbYCgrQ4Uh5RvvXEeFjAFksR9Wf.NhNA
Thanks for this :)
I checked it out and modified it a bit, but I ended up making something else from scratch instead:HiseSnippet 3711.3ocwas0baabEFzwzIhsYZxL8w9vVMsY.soj.o7cZkXaYoZO0JRSjrpU83ICDvRRXABvA.DRLI52R+6zG6Ok9VeL8b1K.6hKjTx0SQhGQr64b1y9ctrmcAvAQgNz33vHiFsNZ1DpQieayCmEjLZ6Q1dAFu5EFM9CM2y9LufgjcscRBilQNzIJz2+.6.puwymMwNNl5Zznwm8WPNZrxMMXW+6u64191ANz7lLLNNzyg9ZuwdI4sdvS+qd996Z6ROxarB028ouxILX6P+vof18YMsLlX6bl8P52aijcilFuzNdjQia2zw4tOh9vA8dfUuGQum6cOsWWK6dVO5QOfZeOKmSeD0t2fScrLZbqcb8f4vgI1IzXPnOOzc1giBOOfO.G6E6cpOEuoqwgvHya1X6Qd9tGHgpXCiF27fbf6y3.2uu4ddtdYsmCfeEqCRNGpfViaLOUp6UPkZnnR2jqRecSvV4MIIuGTe9MMeUPBMZfMXaTUENsF23+rQysCAJBRVer8Yzcifax3vrqkkUGx8srZ2uUKv.EmP.EOAbPT7KHaQbhnvLRoMyUOBnSoAg+zpcHuCDZG7e83+68cHODEuWfuW.kLXZfShWXPExL.7E5Prg16PhYs+b6n+lmaxn1s94VqrwFBu0SsiPCvDZThGMt0J9gN194brmczPu.Pq60uTeGElX6ezHOmyB.LDnQebH2onXxDgK018Pvokpxjt35SZwzxayuHIi7hIwT9zMLveFIZZPLY5D31AdQ.Xyv.rWAK2lwu2.wHPlvve2PZLIHLgPuvKNoi.4HdIsVAH0TZeGRS1NbLHb3FyUWElKHfB+Y0L8kgyq1lr0VjoAtzAfAwscqU.vULKu.lcnA3cVuueViyjM1UowykM1SowQxF2j0H++gYzdP3iXNYCA.DvUKAZhFwmgY7qqnfvjyMaWWge2blWcHWzgLqMNx58rdLEPjyQKLPz40RwHp2vQIq1YTsTX66Gd911nOnyYwfvV8YfYJugZYL1Nk9pfChnvc.aCr8io0P7e+0zTbl9bPhUJPW6D60GcXtiJZ0rpivzpHjaVNZDXJvbOBGMufXOWJ32RUMVnAkvYXhOF.LCRhyLgfkgjDRNURN0EbygFXN9bQx4yKgDOJbJLP..38SfLmlDNFb8AiNDV.rnJyL2Aw8KmyfJwptBpsq6HfY+pgDomv7n4zg70y.p3+HF.7nyFFQqafm.PYPdPJ59LuvzpDhz4fkHmSBGhOjBRGSKvwc.RcirOWXXfLlwDyynzIwn0cLXl.JlztPf29ozHe6YKYbmf5xgchNzA6BtlCy6qccrlYDphWQm0x7UHRUiuqgMpjTjFom4et8r38CNJbBmRzXco5ZDYW5KVvVm.9KHFnFMGPY7f.QExasBSJ.RvhVcjpa4UCKF7r7qR.Jb4jSGDF6gpnoXUhNxUF5HWMnibE.T.slejb8JiVzb6BKjW1OcQyJouJVHBBbwPwJTHYz.JXsgTZP3RADyNvUYMeX4Kso.Oq2TnXKxfvHBVZaPBvuz+pkdfKKQbIShdC8qhGtF3XiUcjoM8EV+Xg0WTrPn.UXIfoEmOrzt3zvNFKcfLxNFx8aGLj515iHt8iIrkMIzfUfer3y3Lma9pSgCzIKyqB6c+Aaq6boghniARUl2QrINz3.yl7hvGUc.ApSsiYKlITfr3Kx4PwdiXf2oTZ.AxOh3GVFVEJy59zfgX0qq7yxzzRa1DQbDatorDLX7r5XwFYwBrxJjpP9P7GGxu.PawxTygpYYTEigK4T7LHf0TED5TWIthEbtDMdU4u5aGmvqjtrcHyuoecb9RlyQkrJ8a5WZbEyEkfOSd3njBQuxPejhgQgP8uPQ3PXCF+pg0jxCvAPwpI+P3Tv4jZJ2Ai4PdoyD3Z35nrfUXLKWJ.AU4KUb3GOYZRVTaD5EFS7FOl55AqeCkCEGRRs8mBsxJ3G7zFfYqFGB4avkDXo4EhgWb2OvDhod.WGhtIMK+Qlp3E.9f19nCuXmFfkfAHbIAghJa6JymMtXXuZAovBDhhLqflzhzTkmPIAUljJjStZB08LbHdFGwrsgWoh3E+BAUuTv0V7Zx6u.xOdYHG0f8GL.bbda8XQNQmjMIXSClyFIh6swrF4oyYExsFXs.R3Inx6SH8JxIWo666z25amh67UwgQ2S58RGeLS.24mECh98lVWr6t6Xg+G33CSlWyhnGFAqKk6WgafOOLrkPLnELgNgz8wBH.mZiBi79Ib2h9J7lDALSLOMLA1EAYLScaqFH9CPcTlui2BdY0Ak9aIxpWx6AK6ByvvS8X1F.2BGIvZkAFPVmLGYw3iIJEDD3gKOSn1iIxXZj.RLKnLWNK5LIPYw0WNOhJsTwvdpXXJdPINUgfQLqyR.fkmZKEJUEhaUK9sn4cNHNW6WdUaY3zB.8J.vMUAPti1ZbzJm6qFfk6KrbZuBYyssZmB28wjWfaATO6AmlTvGPjm8PL8+VkT3aSLK1zF0ujNN5R4lVobylhJBNqsMlyJ9RQmM4x8hc3q0lOzYNLX1nHLsJjN5grq9UP0yfDgoTUZuG6REJeYdBnrSerbZO1LplUV9tpGvGWPakySg+DlYj5htUvZ69zR9VEWprbzxGURtM5ov+d1IiVer8ElJtLcH8rZ2otf22K5Yk6QXk7Dgm0Gpxhl07VOVle5JBvG+IEfuRY6zfqbcMc4rOEVItBfOs.v+dEhJBw7Vy6Gm24ketGqJRXuOtX4cEK1qToCLxyJrNq1gZ1rfLOcskVHJffUDad0y31mXMstiOrQC1YRis+y4ShOUIr9DlzpfxCy92JE8BCd6Wl4SpRuVrmolhjCzyHeqPpey2PjM8DQS2onTyn4BAauUsomHZ5NpVHk5YTLi5gGUUEdRzTZ+5XPuvZ43uVkfphPtrnwHU0XbkBz6WVPmnYUSmuUsJaABpokA0TAnVmsXlfsBlvTgIL85ZKN9pZKNIyVLSyVjVisPyjjCCn.KG3i8OGOl5mZn4Ifd9KU2pXUdLJ9T0NW01RJKoLaa6lZxuCVZ6BeTMsKLHPJY7AyRhmFwNFxoSbwSwmcbvXxYLasGeadUdrF5RaA6l9pDmTGve7RB7GWF3mUCveRs.eZ8.+wKF3S++IvmVMvmtPfmqHxQTdd60rhq39pLrcpbTUgAV+QT1oIXJ6nhHS1IO8lIkCNmepb4IjrbIaTo9xrJV3GzaDMYZTv9m9AnZMfT9oOT7AVnVvGGVPhzdPB.IZ3XtTjOffLgjcZJWB5Ae70Ti9qrRqKq30Z35ezp363fx4ZuzGmM+Y0y4zFYHiaj7Wis+brX2XSqRG8p1Q8iO8F00aJezuy4AkhOwm4ybkvUkgVlYm2dG9IghXC5WvmjPve9YLKHrvALqQrxwJqQc9YJ2R5umI5+nJ0ENZ6e4WTjZ0Dx6rcKd7RdRhxmYaAlKeDtYHQAJUNNWnZ+Ek7nNoT63UNaRgtzRoHGdsoZkf2VYlu9yi3LaVFRyI+xJ8h9XNLbMWK8sXLmm8Etif408725Q9.ltvAT5kV2Hl0+B1ORKwhd6DvVwS47jcC4ucQ3qE1bOuKs8pNm2Ek4ALqogwqo9TMqS7oKg3yPg0zfTM4idN..rqzqAVzW9.zGL02W8cdgUkAwcVf8X96nRIWNfSE2MS6K7hK5ioqmRGMLMCRM9RXs5Eq1NKJPrHS1d9s5TuOj5FSpzCq7PM6pNTJP5BdRtsTjoUk3rn3prmyl7reKhpWmZeTyQojTpp3ZlsB7fJX7XO+z4Z6pHjsNYMawxRQXBJEOmnRsKdzPYVyEG88sDKb+fZSXnsxKEkMtqYprGgMVhcv.YhTEuLw7bUxzpTxzkSIOgojoKRISKpjo0ojkKiQwA3sBicYhTrrmzl6l2h4oiuyr4O+daWW7Op7Kd2ZY+tasu1J7tw5Q3+Ru5rd72DM0txJ9ph9Td6zvC1dS1E56kSTsOUatEH+YZKXYnlbyB7Y62a53s8hb7o3lc5ZIbkwdbXMiYfwiabKhprxWrLmegPwcfYh76wh.f+7DUhHd24NE8VxX4CbV9PAV9fjEc1jJJtxhG30nqv2ov8afuUwEYEeSj9v0i0HaWuoHnUj1MA9Y4mi8BLQ8xZ86AM8A9uZWZz1fbeAzoJdf4iwBnk6dNWXalIrMaWVsNks1u3cjluuabKDlc68PrHeoTuMoauGTA6NJOREK1E4WHlJB8IOgz89sK23Cw1xapvLR8AB3HNRe89QO1c788lDSMeGdNOb7sCYlxuEX9sI8T+86UD1kxZMUBXT1mb9kx9X99vD59Alrsw0BDPwtFLnx9Da4wmFUY23mQQz7XzDbwOkForQIFgFMto92hvsVtuEAQVKEBCCdUfWx9Snh62Mz2E+FCveW9KWvPjZC90ad0Kfzx3Gyfb87r2YensWPS8bn7OsgUZ9BZ7YIgSXzJxGZz3ySX89kxO7A1GohGLzeSyp+5CzWUv3BiFMa3BZxL4OXIbva9mvM7rmx6zeeQMZ76Zp89hZz3FJZ1WTml8mpQyTWL.0q7uhlYp2HzOQCi9GOUpi4sHy+Bscq27l27qXyEdmUWZ.RCsqcN8EM4Q.L89Vp58sJp225e847Fxz6rVT0aXknp06kB9LVFk9OOWDPb3NbODCoGhwGmGx00JXT9a84qZtWn6Te6D8O8H7ixRzAjCP668A+ldBfxkmo9QaU76Q5l0mCvRIGvBhvWR08qadfWhynp02aTg9BYJ9Tquhutqur4NCFPcRxU1a1b22N2Okq+2nJ7hsFBKnF4g9de+zwGBwDNTPSB.egXzW6FXVT98VR2xCoAtra9U3RzYW79FhN6J6zXrM3d8iN7UGvukruf0BnSAru0tUZtGdOoqAaECU7ermq2O53nKpRL165x3lWWFu60kw6ccY79WWFev0kwGtXFwu7vmI9vYfvICi8NXG1RrMZrS.drELOWi+Kf994+b
This one uses a non-opaque panel to draw the scrollbars and to receive the mouse events which move the "contentPanel" position.
I put it in a factory method so hopefully it is easy to make multiples.
In my case I don't need the scrollbars to disappear, but I like that trick with the timer.My main issue now is that I just wish there were some way to also include the scroll wheel/trackpad scrolling.
It seems at the moment there is only solutions for either x and y scrollbars (that remain visible) or use the Viewport and use just the hover scrolling with the wheel/trackpad. -
RE: Keyboard/Midi note triggering button
@cassettedeath said in Keyboard/Midi note triggering button:
1073.3ocyW0saaaCElx1ZXVcqXcnXXWJTLfo.TDXuk1Mfhf5XG6BiUmXDkls65XnnrIBEoFEkyLJx6xdj1iReC1NTR1VJ0Iy0Hsc5hfb9imuygG9Q5wJIgljHUHqlmNOlhr9Ba+4B8zdSwLAZ3gHKa6KkWh5NOFmjPCPVV0egwjUyFnru297tXNVPnqTgPmIYD5KYQL8Jsi67KLNe.NfdJKpj260YHQJ5I4xT.F0saghwjKvSnGgMtUyFY8Y8CXZoxWi0zDjUitxf49SkWJx8+LVB6bN0HzF4CKTt5ARdfAwFsndSY7fwKJ2DDTXiWU70yK9GZOhEvVpeUS3qxL3tJhx8CqZUgW8JvqcY30pD7VCjrJAoF4P5A19DEKVuxhAO2ydnPSUgXnsWFJ49hp880r6IAOD5civWPGn.gkQ38zVsdrK7mcdliihNwkNCbjE.RKBZBU2SFEKEff2i5lp0RQ6GsytIF8BsRx6g47yg8IOoH27dEFLKJSvYBpaXpfnYRg6BedRgOdjEK9icmg4ozcbdiSSVnqWgjSFjFF3tua133twb77ijZC1MP2jDJOg53jaV.lNNLr6794w4UDuwuqdVIbXViiEdKy2HnmBiZlB1X5nznyoJuc1eeHGtNMa5V78lR+e9WQSwzRNy.Zu1PxtImHSwhIz.uJtbkyUNtWGagg4f6Z1JZbbC3ViYyIJ0sEnmHqxJ2tMNBiYUmcsu4Y2xGsH4yIkbTJFJX5iioha5.GpX3xLmWfJvUc1T98Klxy6WHFLL2ztn4gxfbY1EzqFdHViWrPvZB4IlpzLSIXcHcFv9je7oo8gzjKzx3LeKF5fxbCR6etLk+UXm4KE98K5vRFIif0AqlWTru64VfwPFjxw5pzHFtyBCv9SkytlymhDlddYt0qyszXy3VtcpuMEtOvdLSSltd7VaM3E1Q9Pi2Bl5uzteXHknWA1F1C9sOLzxkS+8yS+8r8ABtLtmrj+MYxt+JdF08ETAUYZjsukaM+6M8Vy3M9VyiIZH8mpvhjXYRkE1mFwNEF8SJq7UIv8Bz+3DyNaY88jX0ZM81mO.Jx0FiOVmpxFQNHRlJzUFIpuwiDuG2d23+c2d+wiD3t4AFMWfQeVTLm1WLixAVzLL90.sYHNkqWns5r7HoPFOUJXjxazmP0J1jITUYru1B5.sFd5vJMOryITNEWdn8657RXXCqf9DcK6Eseuer0Z2u9V6b35ZN.59IYe6Ngvt9FQXemfwOEjzmHS0LwjQXXHDt51FdJmOvMRnP1EB3whfNqZF9sb4VF4bxQQPlv+.eEFaajsJL1dgwOJ4HBSTxWSxefjYN8yyz.0sH62C0zdjQ1cMuKJBX4dMgTcodm.+gsMvebaCbusMvmrsA9zsMveZaC7m+uCzbIzAoZYT9QSDZz39Yui0xpu.CS4YmHP+KzWV2PG
This doesn't seem to be in the HISE snippet format:
Make sure you go here to export your snippet:
-
RE: Keyboard/Midi note triggering button
@cassettedeath Try wrapping the snippet with ``` on both sides like this:
-
RE: Printing Viewport view positions?
@d-healey said in Printing Viewport view positions?:
@VirtualVirgin The viewport's changed callback isn't called when moving the scrollbars. It's called when the viewport is in list mode and you select an item in the list, or when you call
.changed()
I just tried attaching a broadcaster to the the property, but that doesn't work either - smells like a bug there @Christoph-Hart
The only things I can suggest trying are a mouse broadcaster or using a timer to poll for changes.
Thanks, I think I'll just leave it alone for now.
I was hoping to use it somehow as a workaround to get scroll wheel events since the panel mouseCallback does not have this data.
And that I was hoping to use as a workaround for the fact that the Viewport obscures the scrollbars when the content inside is lager than 2 dimensions.
So I think for the time being I will have to stick either a panel with scrollbars (and no scroll wheel) or a viewport with scroll wheel and no scroll bars.
Otherwise I guess I would have to learn how to edit the HISE source code, but that seems like a steep learning curve for me at the moment. -
Printing Viewport view positions?
I can't seem to get the control callback to print the view positions of the Viewport dynamically.
HiseSnippet 859.3ocsV08SSDDDe2ROzdpwOhO3iW3ohZvqUPnRLVo.ZiB1XQB9DY6dSoa3tcub2VvFi+O6+A5r2cs2gsBXi1GZ5ryW+1Y9My1NQJNDGqhHT6CFEBD5ss5NRpGzZ.SHIs2lPuq0gB37PUj1QCwZxViBYwwfGgRW3sFinUJSR97iWuEymI4P9QDxgJAG9fHPnyOsSy2K782k4AGHBJX8pMayUxVJe0PDPKX4RBY7SYm.6yLlUxh7NV7.B8wVb9pMfM5Wec25Mf07VsW8Ztr5tMZrNvVyk2qAvp2uG2kPWbGOgVE0UyPziAcKk2ntCTmKSSvghXQOevHTizEyb5wjVCD9dcFWchIDZ4N40pERqUOzZOgmXx440r6knvI2ihEMZoKCR09KfDs.jJmBo6a0kGIB04ZL34VVskZHpOC6MEgRpsjR2njUKEZgTuR.6TX2HTXhGUegq6ScvuVdSaa6m8rDZfPdhyYY7BaroEqcNiE4LlpTy4UNii3IftkJHTIQgpKMwhkLgahzJwFqj5HkeKlueOruWUIGqNSC5gP5KjfS+gRtVnjNSYSU93b8TDQ9Cgks+lcETYrxGVILRX.gA4NgpXQRL95KcVx4I4X2f3Ta5jYxQKsLl6KOJitxn7kjn78MsKf98UZ3ixpIfz961N+tp98motrKqODMS0lwpnKywpxgA8fnhkHigHM5hbyEudbSdZqtfgJYaoP+wPHSdWkumgyY98zLYRFWwvoyPHZpNgQ+fLFM3MtzRDdFJ8jJMI4RTboC4ys2loYiCGFYLagPjVXtTzsgyvkRoCLUr1FhOUqBSrMi5fW7qcx+Zdh2u4nbg1MOW3owsUVzlTBY.HNYf1H8HpYE3TSt3NCk2Pel9hKRLqXyTfcvKL8ZlPkH0ZTwUv+91kx+4NnagN3Uzetlv89VcDZ9fYi2Ry.uXG5+Mdy1UeGqc52G35bvV1Z2itzEy+afxmTCMaK2ioiDHWwZ+gAcw223.hDoD7iMbhRlYfTYWiroxzEjdIB+D+jorlQllor1Xkj.FORcLOcxw7xvMSNAwjL4kyJV6YjclwnR.9P0wb9EC0TNVedc74yqiqNuNt1753KlWGWedcbiq1Qy+i3MC0pfzwIBYuN6jrZiR2QxP1XByk7KbJLvP.
// testing viewport const var Viewport1 = Content.getComponent("Viewport1"); Viewport1.setControlCallback(onViewportControl); inline function onViewportControl(component, value) { Console.print("view position x: " + Viewport1.get("viewPositionX")); Console.print("view position y: " + Viewport1.get("viewPositionY")); };
Is this not the way to do it?
-
RE: Viewport scrollbars are hidden if child is larger in 2 dimensions
@d-healey said in Viewport scrollbars are hidden if child is larger in 2 dimensions:
@VirtualVirgin Maybe this
Thanks, but it doesn't work. I should have mentioned that I tried that option already. I'm not even sure what it does because I have not noticed any difference with "autoHide" as either "Enabled" or "Disabled".
HiseSnippet 1042.3ocsV0sSiaDEdbHFQR6V0sp2spRV6UNKHvw.KaDaUSI.sQEXi1PQ6pUnUSFONYD1y3NdLzzJTea588AnuS8Mn8L9GryRJvF05KR7YN+8Mm4b9FOPJHz3XgDYz7zoQTjwmZNbJWMo2DLii5uOx3qLOkFqX7wVmwnWEIjJqXhTDDLBKiQ6MMBGGS8PFFK8cZWLZTGk97Weyd3.LmPKWBgNSvHziXgLU4pC59CrffCwdzSYgUrdqt8IBdOQfHAf2RlNnHL4B7X5IXsY0LQeONdBx3YlDxVcnuv2cGG2Nzs81ZjaaGrqSmN6Pwa6PF0ghc8GQbPFKefGSIjCUXEMFB5dBuoCmHthmkfyXwrQATsPazPHyYKi5MgE3MnnVEiPF0GTV4VJqx8klGy7X2rdYE7ySUXU5Q0hlQs6BRs+HfjQEHUOCRO1bHQxhTkZz34SL6yUToOFNapBkLaQ098Zl8DfEb05g3KnGJAga7v94NNqYA+zZ2lMgymXk0.LmFz15qsJ7ZLU0SDFI3ff8SyT+Tv9r2VOlpF.0F0qEIPeE01OgSTLA2dbql+ZyFWhkVXHZpIrXcnNRPvA6IR3dw15rlZPBmoFx9EJXm61vZMaLVG2rtE6r+hWOfMdhhHj3.seiW2GZzdMknrwZz2XiM7j3qfDQsjhqrB.vDO+.MJ.Z8zwvWHsrYPVc10hY8RK76177MJ.Crzpq1pYCXS.QQG6iz6Ondgem64qAN7rRfu5MudGJbasaCXuc87Rr68l3O97kB0MOOMw5zdcqcKNcrD7SDJ5q31omRMuto0Gpx2et5zsEZBCpbtp0S8x6xQadR3HpbMqKwAIzaLD5xmczY4G1nCIqKshgBdenB7pHZt7gh.O8Hg98aOngxay0ib4HDLUkNv8E4CbTuBxRDySOwUH1FktIpxIh9w96iU3hvAQFxVDUpX5Mkw9zKANyr44Fl6SiuPIhRsMeBCV9Am7etLwrtSqJbEySAjolFelABMgpGbJjXJZXAMbik+C1S9SsKWBAcfHloOqdaUZ+pJdSUEyf4Uxv7iJXnzTCovcEyLZBMVMMzdNs3EQD9mz0NiZ4nMO1c+stEHtbkHrDRTkLVsNftMWIvRK7RBvpYot0Wpkq.1OyvWp4D4v9b5LaxOfOu9+dSoSklx6ok6AB2GaNfoHSlOdqMG7BMc+ei27aGej4A99.0aIXqad3atyqB+uAJY2xL9XrRxzsTmjDNDZkIT.IbnQKV2cUSOVmI6TzsMjx8RE9a3IWYasrQtx1EJQgX3KhdOIiLPeW7Joq.Xhm9sJMLOVKaMmo+P3SCdOgLantkitKpiatnNt0h531KpiOeQcbmE0wWb+Np+xsuMQIByFmPniGbPJasgwAbLzMl14h9G.O6WGsC
-
Viewport scrollbars are hidden if child is larger in 2 dimensions
When a child component of the Viewport is larger in x and y dimensions, the scrollbars become hidden until you are at the max x or y position:
Is there a solution to keep them both visible at all times? I would think this is the preferred behaviour.
-
RE: Dynamic creation of components?
@d-healey said in Dynamic creation of components?:
@VirtualVirgin At the moment I think the only dynamic components you can add are child panels. Christoph is working on a dynamic container but I don't think it's ready yet.
Thank you :)
I looked into the child panels and made this snippet:
HiseSnippet 1736.3oc2X0saaaCElJIpq1qEqEX6tcgfuXvooMw1M8mztgk+ayV9wnNqKAAAEzRz1bQlzihNIBE4l8BrWh8fzGo8FrcNTxVR1xIoYq6h4aL34+yG44nCYckzkEDHUDqh6G1iQrticiPgtyZcnbAYq0IVuvdeVflKZ63EJnc4tNtc39dN8nBluiqhQ0bovQqBQYzRm.5oLGOplRVMrGMHf4Qrrl9Un8rJLCw76O+9Uo9TgKKgDg7VI2ksMuKWmPs9x+H22eSpGaed2TRu3xa4JEqI8k8gXeZ6JjdT2SnsY6RQwlxl7ZZPGh0CrccWbI1yaU6YUpsD6IdK1rV0JzZUVZomwnOohaykXzZsZ5VgXcqM73ZopglpYADqYVU5E1ni7LQjCdKOf2zmgKpRZ.dNh7lReOLEQpj0Pno9.PMf.VodBDOcDD+k16v83Com.02yvvIQiz.n0TYCuoyDdUSGdUREd4DRVoBoYhBo6a2vUw6oS3fwymaukPyTsnv9T5PIRVxT+wcrWSBRHzy2kdBaSErXnFkqVoRkG57zJUl8kEKVD1sBzN6.oJF20MGd9NmAp2loWS1smT.KJWJiTk.0iTdEOOCkU6q0vItIocVwRTuQ+lZE0UecrQNxlXn5LU.O.0Zc3T9kmJ4HKZnhKrfiBJqjJlitCKcIUPrWvxHTmsYh15NfCxwTnydK0uOq7rn3FZqnTzv48MZ8xh7VNkyZoYK99hEVXgXuGc5v32xPbcADZYwu4CX.JvE52H6CcAXka0W3hU7kaarzoTkCEhNcGd.FNaKco9qJ6K7BJi67EJVnMZinR0xQ+ELuOucGcaEKDEo87sfh72vb0koQqGWgl9P8cDSOE8rHgenSsgxuozr4eJSvUkvycIBuO6bf0b.Uz7WLatI4Nx9Ar0n99MAGkjkLvfZSlhPoY07t9b2SXdyVr.PtfoEXJfDwwBW.ItwQ4bR5+OP5iRCoSHSuRbcxHqGbFUICGCZMtiK7AryYfAcFea.MtOhcQEVlBC.VyzcwTtNTmsg5KiOhTyePoWuwKrRaY7aNfTkLKJ4LmynBoXmxAXXPqhDycTrKdT0iyW5UfrxriCML.XJUehxAy5P8A1dgNryg.OBG8iKxM.XVCd.55zFGS9W4KaR8qKC3HJdfI6GQuCuZ8NLO8947zK6g4ipc73585qgdO93nhL.Vv9mREuMW3zKNbbZIUF5s3JnUZJTqXAnSGaRnC7Iq7S+bYf4Wk7C+Jws.hYcdpL5.37wPKLbSOLkDGNj5YCccz5NCWm472XGpoddiTzTrfQRrfrboygx1yQponEBzBGg1YbOcGf9YiPuCCa0.L5LBCMzU.HOrnHC6I1xyrYbkM8LRLzxUSIZreMBcYMFYhXQLsFQSy7vldTQaeVYpCzgah13rNbMKlqoY4XpCZG26LWCnftZC4MRi0GWIkkMcVSxynFrEvVd3g8d8C5fCYiGtMCZSw1HHqKe7f4GY9.zNQdI9q9i1NMmdu+mzO8Z1pzL+TWI..sTxtiAGebfQjkF.GXsRltOQrgoa6Vmpvo5l.jk2HUHlgMiJyMcEb3Ne6HS1AzlatKeTBvYCchTrqTy1SDY5hWTzYTVsZkKOb9Tkz2moxkMd8J0koXYQ+tMYpG5bJBhwe4dgGD8kIAWmrGf28KZJlr3Lf.Gg6cC3rWyeAJe.pQIeorRW5EinNfDuL+c1fA6rIVEAtGr.lIvcbxdwI6Iewoz2qyMZd9TBJEaA44d8XhIcaOR7k.vKYECafnZyUrt6fqXggLgCWj5qryIYHF3kX801+Pi8188iCJGc7EDxOsEpv.2.5CQQOlRywDzZc3zqKK5lcErWmEbhV1CtT7v6k.20cRA0WXmodlbd5anGldg4qCCIr7uubzmEFR4C+1xYb4smjKumc1owS6ysVNL8BXLR4YCFlL.UcMbhw.muw40PMphH6Q+UD9rlJa3A51yT7lJfFMUIo38YSdSKm4bSGwG9QFw+yhya8wc3JINqGlJN+P6kOcPUf0z3QtsD0g1YLMhjjweuf6YuizquOUm84KvG4IlAz6HyaFfuKf.lNKL8i.8u1aZbcCw6aWmqc6jeLNUNwHTh8oHFieIn6ZuQqVP2pj.bF6MO3Syy9PhF8p8NTshCmCr2se2FvnItv2anB7qU.MXyFU0rtBtFQfFLgmYweA+hYVEWaEyr5.ljtTWk7ctQs9v2Z51FJPLILuKWA3nLr1o5f1bI3bWtG+cttYM0XJV6lp3iuoJt3MUwmbSU7o2TEe1MUwme0JhuL4J80xtQkMDxN02v7MHKqMDT3Dn4zJ4uA3bJKSD
It creates and destroys the child panels dynamically from the buttons:
The child panels are not saved in the component list and will not be remembered on compile,
so I created a method of restoring the panels by using persistent data in another panel.
At this point it just recreates the amount of panels that were there last time, but I would like to be able to recall and restore more complex data from the panels.
A drawback here is that the child panels do not accept "Component.setPropertiesFromJSON" because the child components do not have a name. -
Dynamic creation of components?
I tried a test to see if I could add panels from a button and I get this:
Interface:! Line 29, column 31: Tried to add a component after onInit()
Is there some other method I can use? Is there a way to do it?
-
RE: How many times is the onControl callback supposed to be triggered on compile?
@d-healey said in How many times is the onControl callback supposed to be triggered on compile?:
@VirtualVirgin said in How many times is the onControl callback supposed to be triggered on compile?:
Right now I am using it to update some things after the persistent values have been loaded.
Put the same code in one of your control's callbacks. You could even add a hidden dedicated "post init" component at the end of the list.
@VirtualVirgin said in How many times is the onControl callback supposed to be triggered on compile?:
I generally cannot change the order of callbacks of independent components in a script.
They execute in the order they are placed in the widget list.
The "Component List"?